OpenAI Assistants: How to Create and Use Them
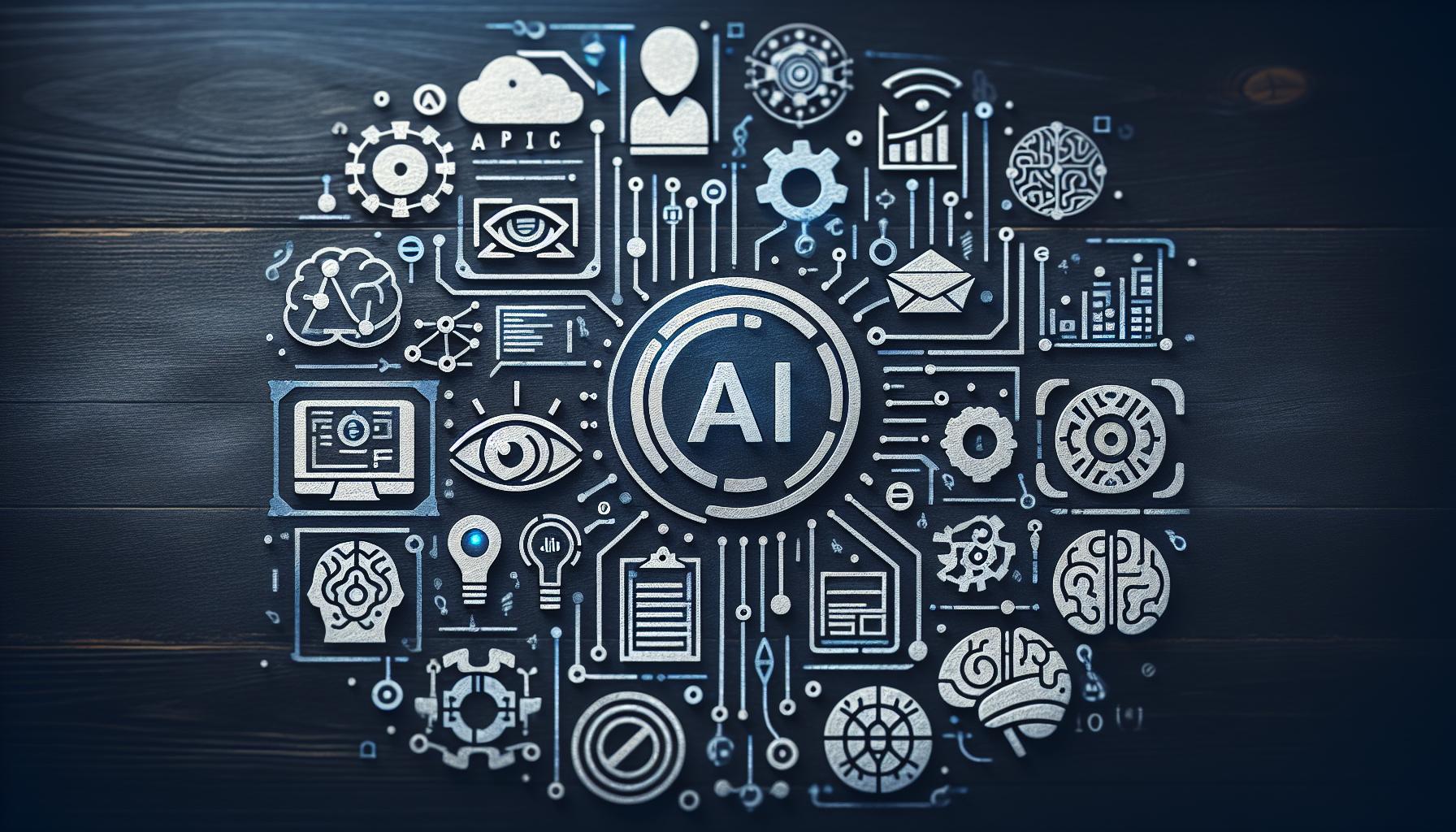
OpenAI Assistants: How to Create and Use Them
OpenAI recently introduced the Assistants API, enabling users to create personalized A.I. assistants. These assistants can integrate your own data, run Python code, and use custom functions and external APIs. This blog will guide you through the process of creating an assistant using both the Playground UI and the Python APIs.
How to Create an Assistant Using the Playground UI
Create the Assistant
- Navigate to the OpenAI Playground: Select 'Assistants' from the dropdown and choose '+ Create assistant.'
- Fill out the Details:
- Name: Terrific Travels
- Instructions: You are a travel agent specializing in world travel. Your job is to suggest itineraries and give travel tips based on provided data.
- Model: Choose
gpt-4-1106-preview
for uploading your own data files.
Choose Your Tools
- Functions: Call custom functions or external APIs.
- Code Interpreter: Write and run Python code in a sandboxed environment.
- Retrieval: Upload files for the assistant to consider when answering questions. For the travel assistant, use the Retrieval tool to upload a CSV file containing travel preferences (e.g., preferred activities, travel times, budget, and visited countries).
Save and Run the Assistant
- Save the Assistant: Click 'Save' after uploading your files.
- Run the Assistant: Enter a message and click 'Run' to get tailored travel recommendations based on the uploaded data.
How to Create an Assistant Using the Python APIs
Key Concepts
- Assistant: Uses OpenAI models and tools.
- Thread: A conversation session between a user and the Assistant.
- Message: Created by the user or the assistant, stored as a list on the Thread.
- Run: An invocation of an Assistant on a Thread, pulling together details and calling models and tools.
- Run Step: Steps the Assistant undertakes as part of a Run.
Setting Up the Python Code
- Install the OpenAI SDK:
pip install --upgrade openai
- Create
assistant.py
and Add Your CSV File.
Example Code
from openai import OpenAI
# Update with your API key
client = OpenAI(api_key='YOUR_API_KEY_HERE')
# Open the CSV file in 'read binary' (rb) mode, with the 'assistants' purpose
file = client.files.create(
file=open('TravelPreferences.csv', 'rb'),
purpose='assistants'
)
# Create and configure the assistant
assistant = client.beta.assistants.create(
name='Terrific Travels',
instructions='You are a travel agent specializing in world travel. Your job is to suggest itineraries and give travel tips based on provided data.',
model='gpt-4-1106-preview',
tools=[{'type': 'retrieval'}],
file_ids=[file.id]
)
# Create a thread for the conversation
thread = client.beta.threads.create()
# Create the user message and add it to the thread
message = client.beta.threads.messages.create(
thread_id=thread.id,
role='user',
content='I'd like help planning a new trip based on criteria for Amber. I'd prefer to visit a country I haven't been to yet. What would you suggest?',
)
# Create the Run, passing in the thread and the assistant
run = client.beta.threads.runs.create(
thread_id=thread.id,
assistant_id=assistant.id
)
# Periodically retrieve the Run status
while run.status != 'completed':
keep_retrieving_run = client.beta.threads.runs.retrieve(
thread_id=thread.id,
run_id=run.id
)
print(f'Run status: {keep_retrieving_run.status}')
if keep_retrieving_run.status == 'completed':
print('\nRun completed')
break
# Retrieve messages added by the Assistant to the thread
all_messages = client.beta.threads.messages.list(
thread_id=thread.id
)
# Print the messages from the user and the assistant
print('######################################################\n')
print(f'USER: {message.content}')
print(f'ASSISTANT: {all_messages.data[0].content}')
Running the Code
python assistant.py
The script will run, periodically checking the status, and finally print the user and assistant messages.
Conclusion
Creating and using OpenAI Assistants allows for personalized, powerful interactions. By integrating custom data, running code on-the-fly, and calling external APIs, these assistants can significantly enhance your applications.
Additional Resources
- Getting Started on Prompt Engineering with Generative AI
- OpenAI Assistant API course
- OpenAI: Prompt Engineering Best Practices
- Developing Generative A.I. Applications with Python and OpenAI
- Using ChatGPT to Code a Full-stack Web Application
By following these steps, you can create versatile and efficient assistants that cater to your specific needs and use cases, enhancing your application's interactivity and functionality.